Buttons - Android Button Examples
http://www.viralandroid.com/2015/09/android-button-style-examples.html
Button is available in android.widget.Button package. Let’s create these Buttons step by step.
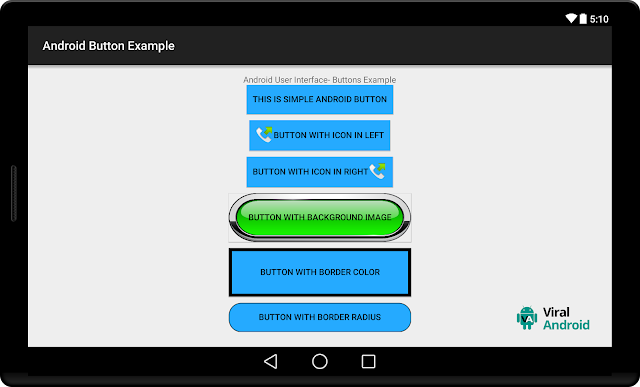
Android Button onClick
Simple Android Alert Dialog
Android Toast - How to Display Simple Toast Message in Android
Create a Project
Let’s start making these buttons by creating new android project with following information.
Application name: Android Buttons Example
Company Domain: viralandroid.com
Package name: com.viralandroid.androidbuttonsexample
Minimum SDK: Android 2.2 (API 8 Froyo)
1. Simple Android Button
First of all, let’s create simple button. This contains little text, background color, etc. Add following code to your XML activity layout file (main_activity.xml).
To handle button click, you have to add onClick attribute in your button and give a button name like simpleButton.
To get response on button click you have to add following code to your java activity file (MainActivity.xml).
public void simpleButton(View view) { Toast.makeText(getApplicationContext(), "This is Simple Android Button", Toast.LENGTH_LONG).show(); }
2. Button With Icon In Left
Like above, add following code to your XML and java file.
XML Code:
Java Code:
public void leftIconButton(View view) { Toast.makeText(getApplicationContext(), "Android Button With Icon in Left", Toast.LENGTH_LONG).show(); }
3. Button With Icon In Right
XML Code:
Java Code:
public void rightIconButton(View view) { Toast.makeText(getApplicationContext(), "Android Button With Icon in Right", Toast.LENGTH_LONG).show(); }
4. Button With Background Image
XML Code:
Java Code:
public void backgroundImageButton(View view) { Toast.makeText(getApplicationContext(), "Android Button With Background Image", Toast.LENGTH_LONG).show(); }
5. Button With Border Color
At first, you have to create a XML file in drawable folder called button_border.xml.
res/drawable/ button_border.xml
XML Code:
Java Code:
public void borderButton(View view) { Toast.makeText(getApplicationContext(), "Android Button With Border Color", Toast.LENGTH_LONG).show(); }
6. Button With Border Radius
Create border_radius.xml file in your drawable folder.
res/drawable/ border_radius.xml
XML Code:
Java Code:
public void borderRadiusButton(View view) { Toast.makeText(getApplicationContext(), "Android Button With Border Radius", Toast.LENGTH_LONG).show(); }
7. Round Button
Create round_button.xml file in your drawable folder.
res/drawable/ round_button.xml
XML Code:
Java Code:
That’s all. You have done with each styles, following is the final XML and java file code of all buttons.public void roundButton(View view) { Toast.makeText(getApplicationContext(), "Android Round Button", Toast.LENGTH_LONG).show(); }
res/layout/ activity_main.xml
src/MainActivity.java